App Actions
Built-in outputs for driving conversions
Introduction
Actions can be referenced by your Javascript to invoke an action in the mobile app. This is where you will pair any business logic in your solution with a direct outcome in your users’ experience.
Using an App Action
In the Tapcart Text Editor
Actions will work out of the box as long as you are building inside of the Custom Blocks editor (In the Javascript tab). No further setup is required beyond using the proper syntax, and calling the function with the required parameters.
In a Custom Hosted Solution
Make sure you've already installed the relevant SDK, from there, they can be referenced as laid out here.
Testing Actions
Some App Actions do not return success/error states to the block upon being called. However, you can manually test actions via the block preview in the Custom Blocks text editor or in your own IDE's console. Actions will be printed to the browser console when successfully invoked.
Available App Actions
action
action
Dependencies for action:
Custom Block Code Editor version: 1.13+
Generic action that includes action name, action specific object (even when empty) and any resulting functions. Demonstration of how all actions called this way can have optional haptic feedback attached.
useActions().action("action/name", {
hapticFeedback: true,
anyOtherFields: "additional info"
}, {
onSuccess: (res) => {
console.log("actionButton success", res);
document.getElementById('actionTextField').value = JSON.stringify(res);
}
})
cart/add
cart/add
Dependencies for cart/add:
Custom Block Code Editor version: 1.13+
Use the cart/add
to support interactions that add 1 or more products to the cart. This action accepts an array of lineItems
, and additionally accepts custom line item attributes
. Attributes are helpful for passing custom data back to Shopify, and any other downstream systems as a result of an order. Note:If you use this App Action it will disable Apple Pay on the PDP if you have that feature turned on.
useActions().action("cart/add", {
lineItems: [
{
variantId: 'String',
quantity: Number,
sellingPlanId: 'String',
attributes: [ // optional
{
key: 'String',
value: 'String',
},
],
},
],
})
Navigating the user to checkout
Upon invoking the
cart/add
function, the app will reference this setting to determine if the user should be automatically navigated to the cart, or left where they are.
cart/updateNote
cart/updateNote
Dependencies for cart/updateNote:
Custom Block Code Editor version: 1.13+
Use the cart/updateNote
to update the note on the cart. These cart notes will be added once the cart is created (as soon as an item exists in the cart). The note will be removed once the cart has been ordered/completed
useActions().action("cart/updateNote", {
note:'String'
})
Note: The cart only has one note. Updating the cart note will override any existing cart notes. Be sure to pull the current cart notes to see if you need to include it in the new cart note.
cart/updateAttributes
cart/updateAttributes
Dependencies for cart/updateAttributes:
Custom Block Code Editor version: 1.13+
App must use Cart API
Use the updateAttributes
to update the attributes on the cart. This action passes key-value pairs that contains additional information about the cart. These cart attributes will be added once the cart is created (as soon as an item exists in the cart). They will be removed once the cart has been ordered/completed.
useActions().action("cart/updateAttributes", {
attributes: [ // required
{
key: 'String (key1)',
value: 'String (value1)'
},
{
key: 'String (key2)',
value: 'String (value2)'
}
]
})
Note: Each attribute key is unique. If you apply multiple attributes with the same key, they will be overwritten. Updating the cart attributes will override any existing cart attributes. Be sure to pull the current cart attributes to see if you need to include it in the new cart attributes.
cart/remove
cart/remove
Dependencies for cart/remove:
Custom Block Code Editor version: 1.13+
Use the cart/remove
to support interactions that remove 1 or more products from the cart. This action accepts an array of lineItems
, and additionally accepts custom line item attributes
. Attributes are helpful for passing custom data back to Shopify, and any other downstream systems as a result of an order.
Note:If you use this App Action it will disable Apple Pay on the PDP if you have that feature turned on.
useActions().action("cart/remove", {
lineItems: [
{
variantId: 'String',
quantity: Number,
sellingPlanId: 'String',
note: 'String',
attributes: [ // optional
{
key: 'String',
value: 'String',
},
],
},
],
})
product/open
product/open
Dependencies for product/open:
Custom Block Code Editor version: 1.13+
Use the openProduct
to support interactions that take a user to a product detail page. This action accepts a productId
, and an optional variantId
if a specific variant needs to be pre-selecting upon opening the page. The isRelatedProduct
setting can be used if you are looking to relate different productIDs together into a single PDP. Setting isRelatedProduct
to true
will passively transition to new products from one PDP to another, setting it to false
will reload a new page when transferring to a new PDP (Available only on PDPs).
useActions().action("product/open", {
productId: 'String',
variantId: 'String', // optional
isRelatedProduct: Boolean // optional, works only on PDPs
})
collection/open
collection/open
Dependencies for collection/open:
Custom Block Code Editor version: 1.13+
Use the collection/open
to support interactions that take a user to a product list page. This action accepts a collectionId
.
useActions().action("collection/open", {
collectionId: 'String',
})
screen/open
screen/open
Dependencies for screen/open:
Custom Block Code Editor version: 1.13+
Use the screen/open
to support interactions that take a user to a specific screen within the mobile app. This action accepts the type of screen, which will always be internal
if you are using a screen defined within the mobile app or web
if your are using a webview, while both types require a URL path for the screen. If using a web
type page URLs should be the full URL such as "https://www.example.com". CheckoutUrl is optional field when opening the internal /checkout
route. If none is supplied then one will be generated from the users current cart.
useActions().action("screen/open", {
destination: {
type: "internal | web",
url: "/some-known-url",
checkoutUrl: "/checkout_url" // optional
}
})
Below are available screen URLs in app you can choose from. Additionally, you can call any custom screen you have added to the app using the method's below. Those with a ? can add params as per standard Url practise
Dependencies for screen/open:
Custom Block Code Editor version: 1.13+
Mobile App Screen Name | Type | URL |
---|---|---|
Account | internal | /account? |
Cart | internal | /cart? |
Collections | internal | /collections?id=:id |
Checkout | internal | /checkout |
Home | internal | /home? |
Products | internal | /products?id=:id |
Orders | internal | /orders |
Mutli-page | internal | /home?tabIndex=1 |
Notifications | internal | /notifications |
Wishlist | internal | /wishlist |
Wishlists | internal | /wishlists |
Loyalty | internal | /loyalty |
Subscriptions | internal | /subscriptions |
Post purchase | internal | /post-purchase? |
Data opt out | internal | /data-opt-out |
Contact sharing | internal | /contact-sharing |
Delete account | internal | /delete-account |
My account | internal | /my-account |
Main | internal | /main |
Search | internal | /search? |
Search results | internal | /searchResults?searchQuerry=:query |
SMS opt in | internal | /sms-optin |
Store location | internal | /store-location |
Product details page | internal | /pdp? |
Products list page | internal | /plp? |
Custom Screen - New format | internal | /phoenix-pages/:id |
Custom Screen - Web-based | internal | /hybrid-pages/:id |
Custom Screen- Block-based | internal | /blocks-page/:id |
Webview | web | https://www.tapcart.com/ |
Note
If you're linking a Custom Screen, the Screen ID can be found on the custom screen settings panel under the Screen Title (see image below)
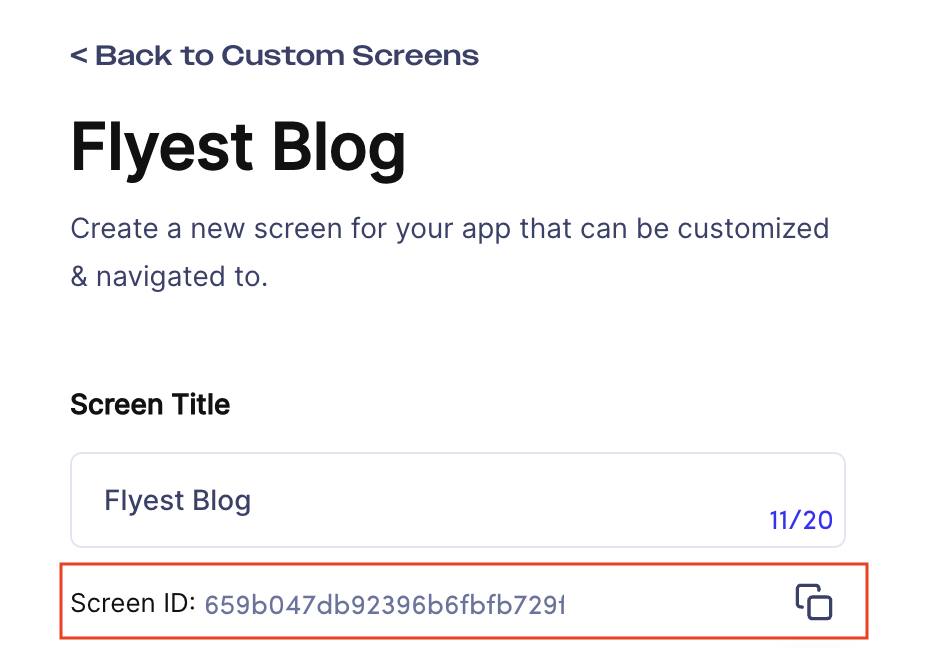
customer/indentity
customer/indentity
Dependencies for customer/identity:
Custom Block Code Editor version: 1.13+
Use the customer/identity
to request the email address of a user who is signed into the app. This action is asynchronous and accepts an onSuccess
callback function will fire when it completes. The customer data will be passed as a parameter to your callback function.
useActions().action("customer/identity", {
onSuccess: (res) => {
console.log("getCustomerIdentity success", res);
}
})
"customer": {
"email": "[email protected]",
"firstName": "John",
"shopifyId": "1234"
},
discount/apply
discount/apply
Dependencies for discount/apply:
Custom Block Code Editor version: 1.13+
Use the discount/apply
to allow a solution to apply a discount to a user's cart. This action accepts a discountCode
. To use this action, the cart will need at least 1 product in it, so make sure to add a product to the cart before invoking this action. When calling this action, the discount won't be applied until the user visits the cart, this allows you to manage the applied discounts using a combination of discount/remove/all
& discount/apply
leading up to the user's arrival at the cart.
useActions().action("discount/apply", {
discountCode: 'String',
})
discount/remove/all
discount/remove/all
Dependencies for discount/remove/all:
Custom Block Code Editor version: 1.13+
Use the discount/remove/all
to clear a user's cart of any applied discounts. This action does not accept any parameters, and will remove all discounts that are currently applied to the cart, or in queue to be applied to the cart from the applyDiscount
action. If you want to selectively remove discounts, you should first clear the cart of discounts, and reapply discounts as needed using discount/apply
. You can leverage the Tapcart.variables.cart.appliedDiscounts
variable to parse any applied discounts before calling discount/remove/all
.
useActions().action("discount/remove/all", {})
app/toast
app/toast
Dependencies for app/toast:
Custom Block Code Editor version: 1.13+
Use the app/toast
to display success or error messages to your end users. This will display natively & will be presented at the top of the page. This action accepts a a message
, and a type
to denote if it's a success or error message.
useActions().action("app/toast", {
message: 'String',
type: 'String', // "success" || "error"
})
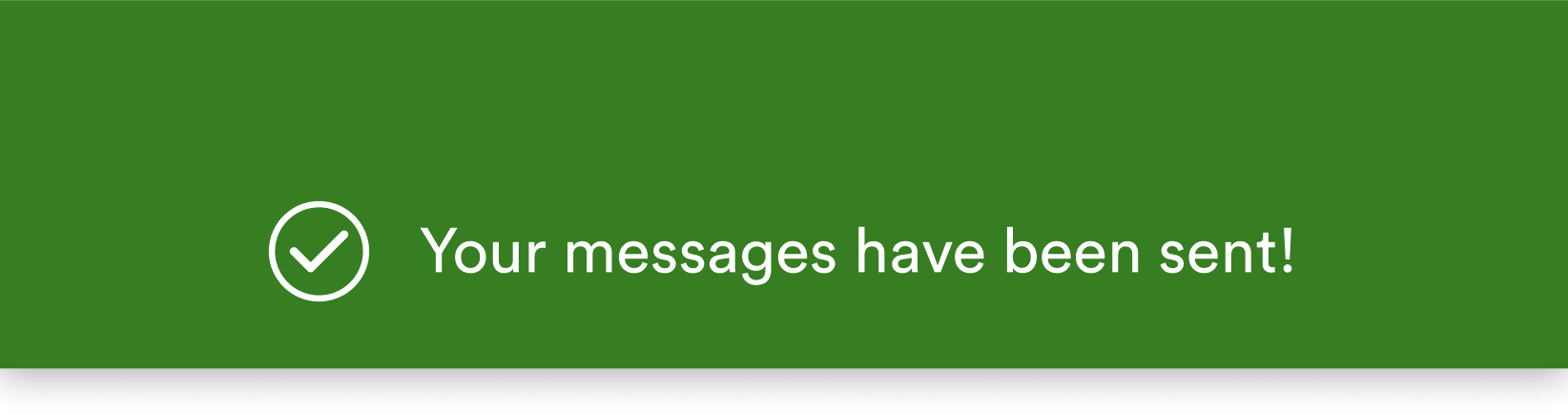
Example of a 'Success' toast
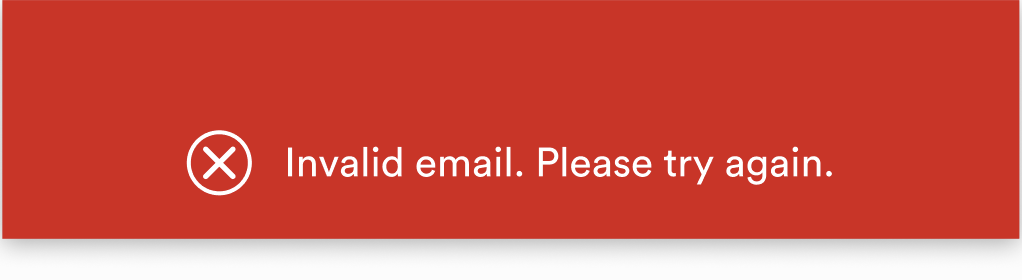
Example of an 'Error' toast
view/scrollToBlockTop
// view/scrollToBlockBottom
view/scrollToBlockTop
// view/scrollToBlockBottom
Custom Block Code Editor version: 1.13+
Use the scrollToBlockTop
or scrollToBlockBottom
to scroll to the top or bottom of a block you've built when needed.
useActions().action("view/scrollToBlockTop", {})
useActions().action("view/scrollToBlockBottom", {})
authentication/open
authentication/open
Dependencies for authentication/open:
Custom Block Code Editor version: 1.10+
Use the openAuthentication
app action to overlay the authentication (create account or login) screen on top of the users current page. If the login/create account is successful then the user will be returned to the page they were on. If failure then the user will be kept on the login/create account screen. If the user exits out of the page, then they will land on the original screen they were on.
useActions().action("authentication/open", {
action: "login" // Opens up the auth login page
})
useActions().action("authentication/open", {
action: "create" //Opens up the create account page
})
useActions().action("authentication/open", {}) // Opens up the auth login page
Note: If you complete the auth flow, it should dismiss and take you to the previous screen. When you do, the console should print out true
indicating the action succeeded.
If you back out of the auth flow, it should dismiss and take you to the previous screen. When you do, the an exception will be thrown to the console stating the flow did not succeed.
wishlist/create
wishlist/create
Dependencies for wishlist/create:
Custom Block Code Editor version: 1.13+
Use the wishlist/create
app action to create a wishlist with the specified name. Returns the id of said wishlist
useActions().action("wishlist/create", {
name: "Wishlist Name" // Creates a wishlist with the inputted (wishlist Name) name
})
Note: The wishlist provider needs to still be entered into the integration object (native/swym)
wishlist/add
wishlist/add
Dependencies for wishlist/add:
Custom Block Code Editor version: 1.13+
Use the wishlist/add
app action to add the specific variant/product to the wishlist. Returns a success/failure.
useActions().action("wishlist/add", { // Adds the variant/product to the wishlist
productId: "product Id String",
variantId: "variant Id String",
wishlistId: "wishlist Id String"
})
Note: The wishlist provider needs to still be entered into the integration object (native/swym)
wishlist/remove
wishlist/remove
Dependencies for wishlist/remove:
Custom Block Code Editor version: 1.13+
Use the wishlist/remove
app action to remove the specific variant/product from the wishlist. Returns a success/failure.
useActions().action("wishlist/remove", { //remove the variant/product to the wishlist
productId: "product Id String",
variantId: "variant Id String",
wishlistId: "wishlist Id String"
})
Note: The wishlist provider needs to still be entered into the integration object (native/swym)
wishlists/get
wishlists/get
Dependencies for wishlists/get:
Custom Block Code Editor version: 1.13+
Use the wishlists/get
app action to get the wishlists and all items in the wishlists.
useActions().action("wishlists/get", {}, {
onSuccess: (result) => {
setWishlists(result);
}, onError: (result) => {
setWishlists("Error");
}
}) // retrieves all available wishlists
Note: The wishlist provider needs to still be entered into the integration object (native/swym)
trigger/haptic
trigger/haptic
Dependencies for trigger/haptic:
Custom Block Code Editor version: 1.13+
Use the trigger/haptic
action to cause haptic feedback in device if the user has it enabled on their device.
useActions().action("trigger/haptic")
customer/session/get
-
customer/session/get
-Dependencies for customer/session/get:
Custom Block Code Editor version: 1.13+
Use the customer/session/get
action to retrieve customer session, including: customer access token, storefront session token and customer session token.
useActions().action("customer/session/get", {
onSuccess: (res) => {
console.log("getCustomerSession success", res);
document.getElementById('getCustomerSession').value = JSON.stringify(res);
}
})
customer/session/set
-
customer/session/set
-Dependencies for customer/session/set:
Custom Block Code Editor version: 1.13+
Use the customer/session/set
action to set the customer session token remotely.
useActions().action("customer/session/set",{
customerSession:{
customerAccessToken: "token1",
customerSessionToken: "token3",
storefrontSessionToken: "token2"
},
error: "Error message"
})
permissions/notifications
-
permissions/notifications
-Dependencies for permissions/notifications:
Custom Block Code Editor version: 1.13+
Use the permissions/notifications
action to open users notifications permissions.
useActions().action("permissions/notifications")
analytics/track
analytics/track
Dependencies for analytics/track:
Custom Block Code Editor version: 1.13+
Use the analytics/track
action to track either "collectionViewed" or "productViewed" events with the relevant properties
useActions().action("analytics/track", {
event: "Name of event",
analyticProperties: {
collectionId: "23466542",
collectionTitle: "best collection",
productId: "3444232",
productTitle: "Big Shoes",
productType: "Boot",
productPrice: "34.88",
productTagList: ["New Shoes", "Winter Boots"],
productBadgePositioningList: ["Badge1", "Badge2"]
})
login/multipass
login/multipass
Dependencies for login/multipass:
Custom Block Code Editor version: 1.13+
Use the login/multipass
action to login using multipass token and optional email, returns Tapcart customer object on success - see customer/identity
for details of the response
useActions().action("login/multipass", {
email: "[email protected]", // optional
multipassToken: "token"
}, {
onSuccess: (result) => {
document.getElementById('multipassToken').value = "success"
}, onError: (result) => {
document.getElementById('multipassToken').value = "failure"
}
})
login/storefront
login/storefront
Dependencies for login/storefront:
Custom Block Code Editor version: 1.13+
Use the login/storefront
action to login using a storefront access token, returns Tapcart customer object on success. Seecustomer/identity
for details on the response.
useActions().action("login/storefront", {
customerAccessToken: "token",
expirationDate: "expirationDateAsISOString"
}, {
onSuccess: (result) => {
document.getElementById('storefrontAccessToken').value = "success"
}, onError: (result) => {
document.getElementById('storefrontAccessToken').value = "failure"
}
})
app/share
-
app/share
-Dependencies for app/share:
Custom Block Code Editor version: 1.13+
Use the app/share
action to enable text messages to be shared from the users device through connected apps.
useActions().action("app/share", {
text: "text to share"
})
app/logout
-
app/logout
-Dependencies for app/logout:
Custom Block Code Editor version: 1.13+
Use the app/logout
action to log the user out of the app, returns a boolean for success or failure.
useActions().action("app/logout", {}, {
onSuccess: (result) => {
document.getElementById('logoutResult').value = "success";
}, onError: (result) => {
document.getElementById('logoutResult').value = "failure";
}
})
Updated 6 days ago